Introduction
In this tutorial, we we want to draw several Plots in one figure. We can achieve this by using Subplots. In order to create a Subplot, we use the subplot() function of Matplotlib.
Import Libraries
First, we import the Pyplot submodule. The Pyplot submodule contains a collection of important functions such as the plot() function or the subplot() function. In addition, we import NumPy.
import matplotlib.pyplot as plt
import numpy as np
Define Data
Now, let's define our example data . In order to do this, we create the following NumPy Arrays:
#Data for plot 1
x1 = np.array([1, 2, 3, 4, 5])
y1 = np.array([4, 6, 10, 12, 18])
#Data for plot 2
x2 = np.array([1, 2, 3, 4, 5])
y2 = np.array([5, 4, 11, 13, 16])
#Data for plot 3
x3 = np.array([1, 2, 3, 4, 5])
y3 = np.array([10, 12, 14, 8, 7])
Subplot() function
The subplot() function uses three arguments:
- First argument: Number of rows of the figure
- Second argument: Number of columns of the figure
- Third argument: Index of the current subplot
In our case we want to have three plots. So we have the following opportunities for drawing the figure:
- One column and three rows
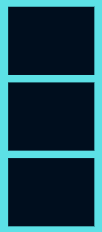
- Three columns and one row
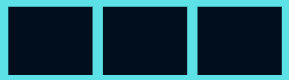
Subplot with one column and three rows
Let's create a Subplot with one column and three rows.
#plot 1
plt.subplot(3, 1, 1)
plt.plot(x1,y1)
plt.title('plot 1')
#plot 1
plt.subplot(3, 1, 2)
plt.plot(x2,y2)
plt.title('plot 2')
#plot 1
plt.subplot(3, 1, 3)
plt.plot(x3,y3)
plt.title('plot 3')
plt.tight_layout()
plt.show()
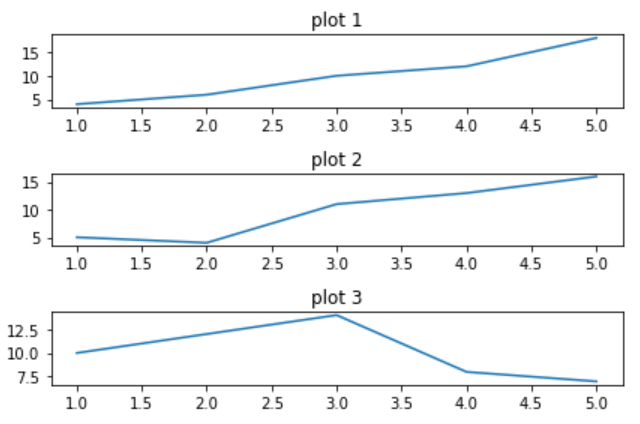
Subplot with three columns and one row
Let's create a Subplot with three columns and one row.
#plot 1
plt.subplot(1, 3, 1)
plt.plot(x1,y1)
plt.title('plot 1')
#plot 2
plt.subplot(1, 3, 2)
plt.plot(x2,y2)
plt.title('plot 2')
#plot 3
plt.subplot(1, 3, 3)
plt.plot(x3,y3)
plt.title('plot 3')
plt.tight_layout()
plt.show()
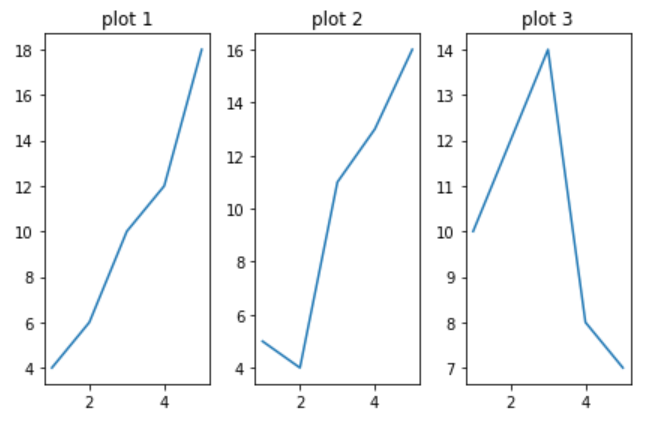
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to create Subplots. We can simply use the subplot() function of Matplotlib. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.