Introduction
In this tutorial, we want to select specific columns from a Pandas DataFrame. In order to do this, we use square brackets []
, the loc() method and the iloc() method of Pandas.
Import Libraries
First, we import the following python modules:
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
data = {
"language": ["Python", "Python", "Java", "JavaScript"],
"framework": ["Django", "FastAPI", "Spring", "ReactJS"],
"users": [20000, 9000, 7000, 5000]
}
df = pd.DataFrame(data)
df
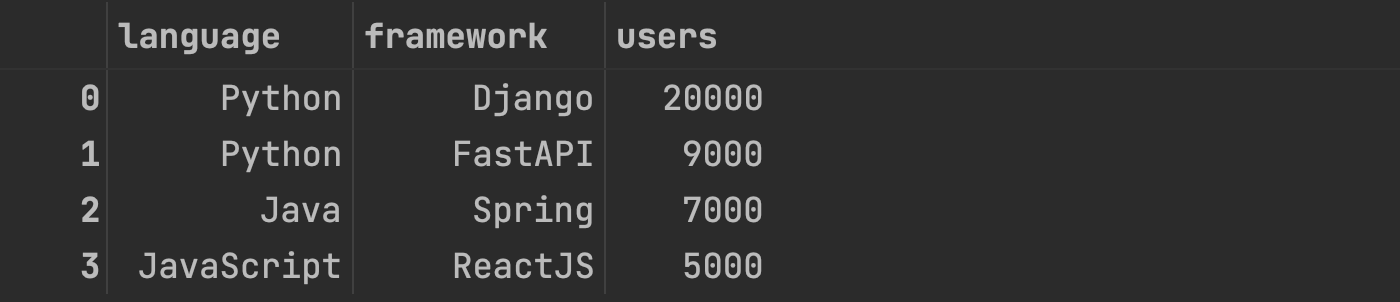
Select a Single Column
Now, we want to select a single column from the DataFrame. In the following example, we select the column "framework".
To do this, we use square brackets []
with the column name:
new_df = df["framework"]
new_df
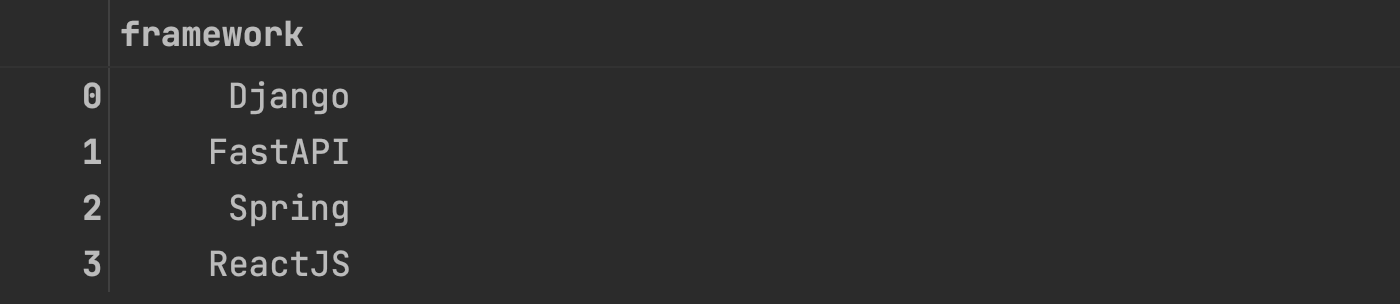
Another way is to use the loc() method of Pandas:
new_df = df.loc[:, "framework"]
new_df
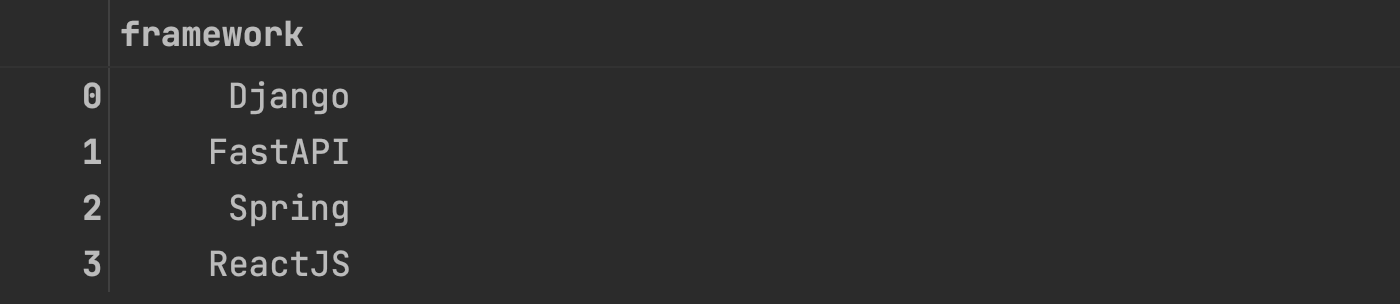
We can also select the column by index using the iloc() method of Pandas:
new_df = df.iloc[:, 1]
new_df
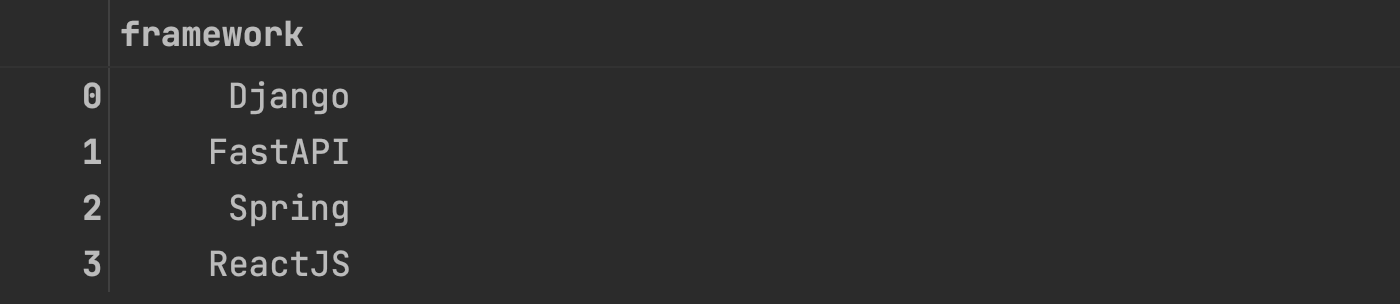
Select Multiple Columns
Next, we want to select multiple columns from the DataFrame. In the following example, we select the columns "framework" and "users".
To do this, we use square brackets []
with a list of column names:
new_df = df[["framework", "users"]]
new_df
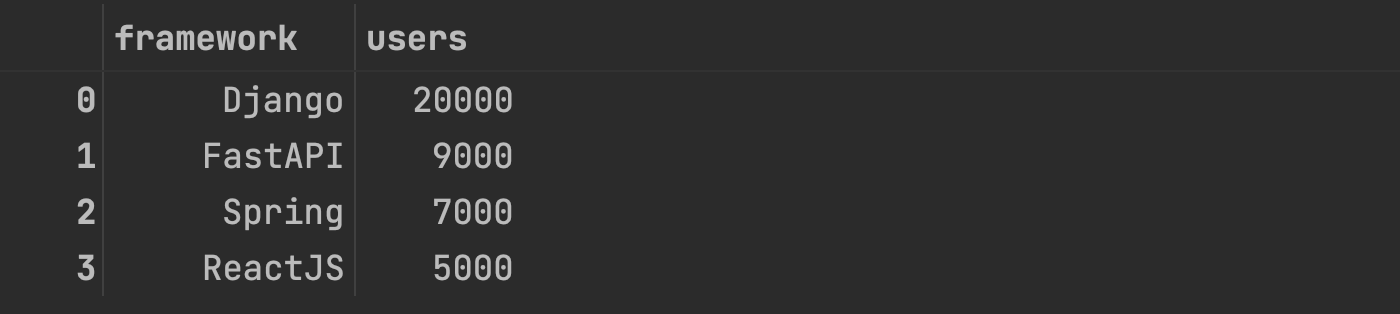
Another way is to use the loc() method of Pandas:
new_df = df.loc[:, ["framework", "users"]]
new_df
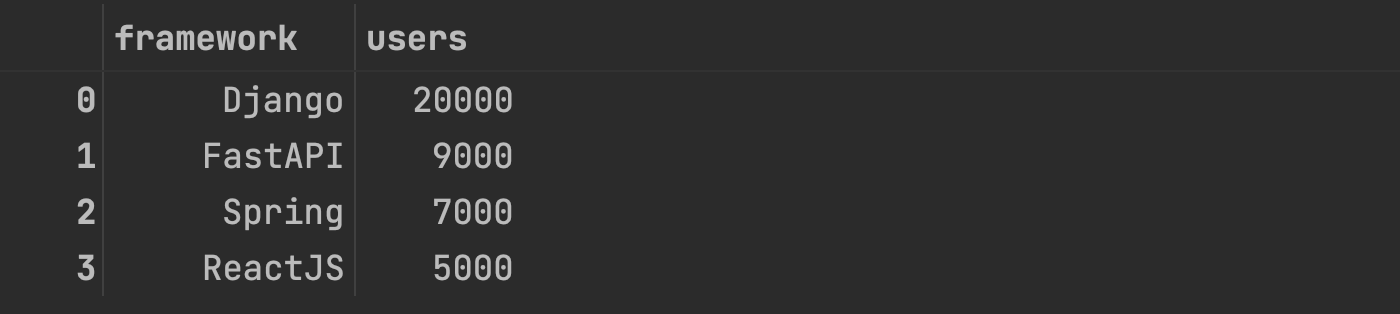
We can also select the columns by index using the iloc() method of Pandas:
new_df = df.iloc[:, 1:]
new_df
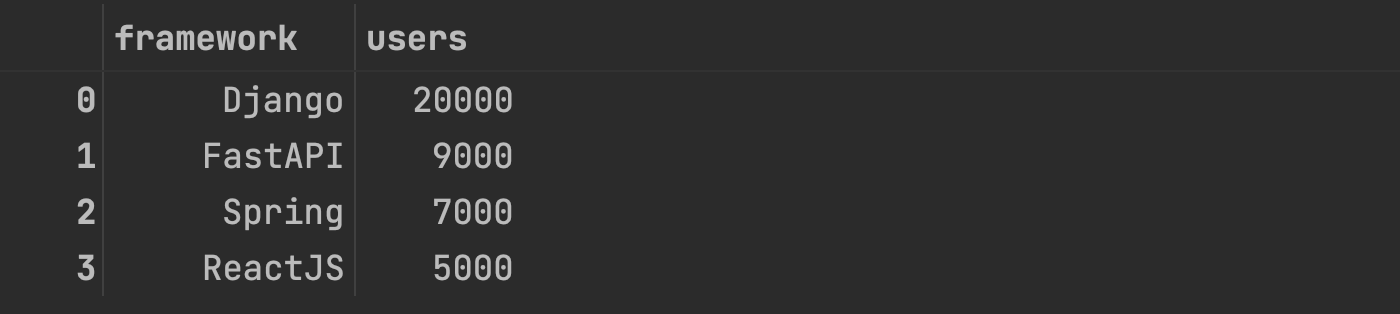
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to select specific columns from a Pandas DataFrame. We can use square brackets []
, the loc() method and the iloc() method of Pandas. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.