Introduction
In this tutorial, we want to rename a Pandas DataFrame column. In order to do this, we use the the rename() method of Pandas.
Import Libraries
First, we import the following python modules:
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
mydict = {
"language": ["Python", "Python", "Java", "JavaScript"],
"framework": ["Django", "FastAPI", "Spring", "ReactJS"],
"users": [20000, 9000, 7000, 5000],
}
df = pd.DataFrame(mydict)
df
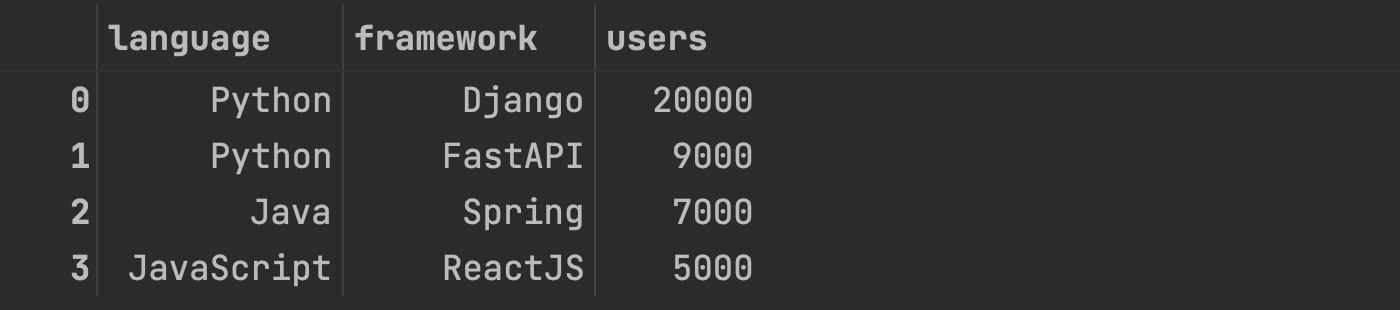
Rename a Single Column
Now, we would like to rename the column "language" into "column_1".
To do this, we use the rename() method of Pandas and pass a dictionary with the existing column name and the new column name as argument:
df = df.rename(columns={"language": "column_1"})
df
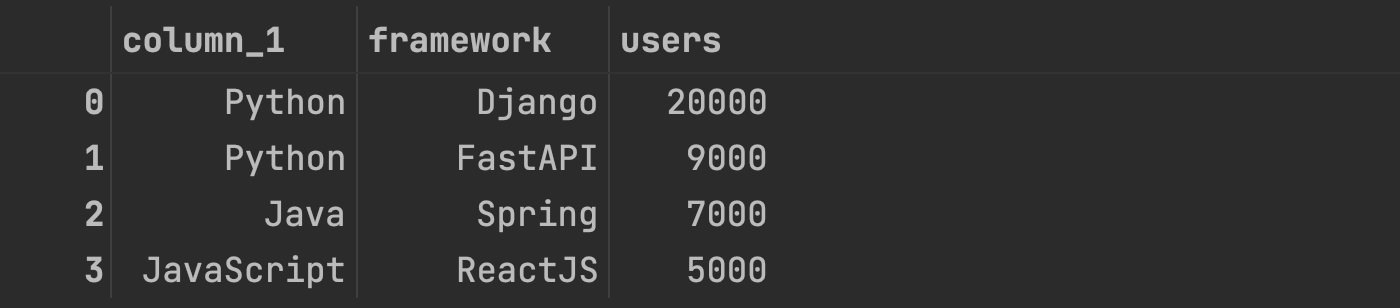
Rename Multiple Columns
Now, we would like to rename the column "framework" into "column 2" and the column "users" into "column 3".
To do this, we use the rename() method of Pandas and pass a dictionary with the existing column names and the new column names as argument:
df = df.rename(columns={"framework": "column_2", "users": "column_3"})
df
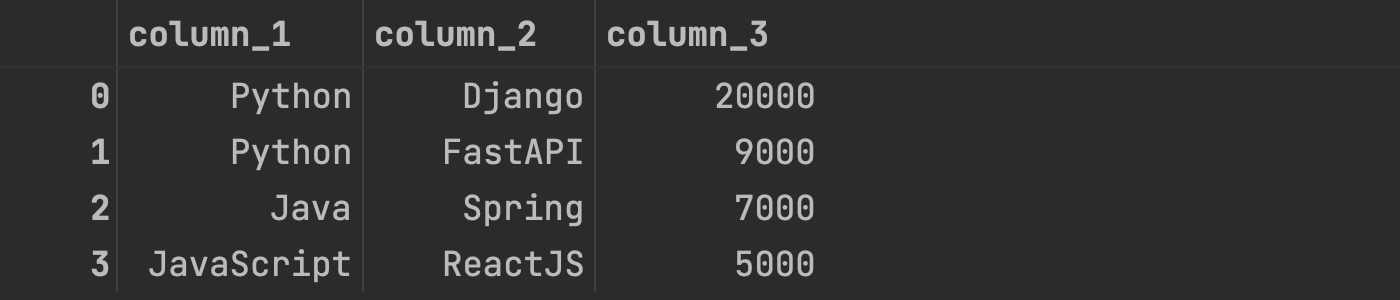
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to rename a Pandas DataFrame column. We can simply use the rename() method of Pandas. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.