Introduction
In this tutorial, we want to add columns to a Pandas DataFrame. In order to do this, we use square brackets []
with the name of the new column.
Import Libraries
First, we import the following python modules:
import numpy as np
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
data = {
"language": ["Python", "Python", "Java", "JavaScript"],
"framework": ["Django", "FastAPI", "Spring", "ReactJS"],
"users": [20000, 9000, 7000, 5000]
}
df = pd.DataFrame(data)
df
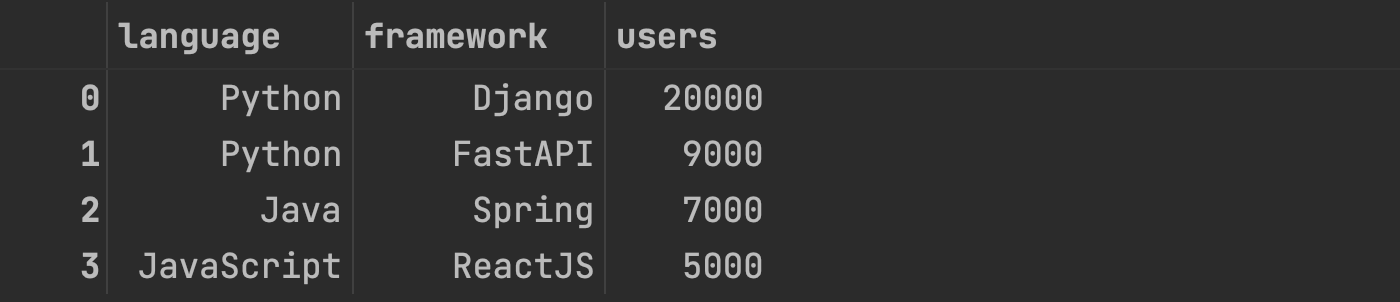
Add Column with constant Value
Now, we would like to add the column "country" with the constant value "Germany".
To do this, we use square brackets []
:
df["country"] = "Germany"
df
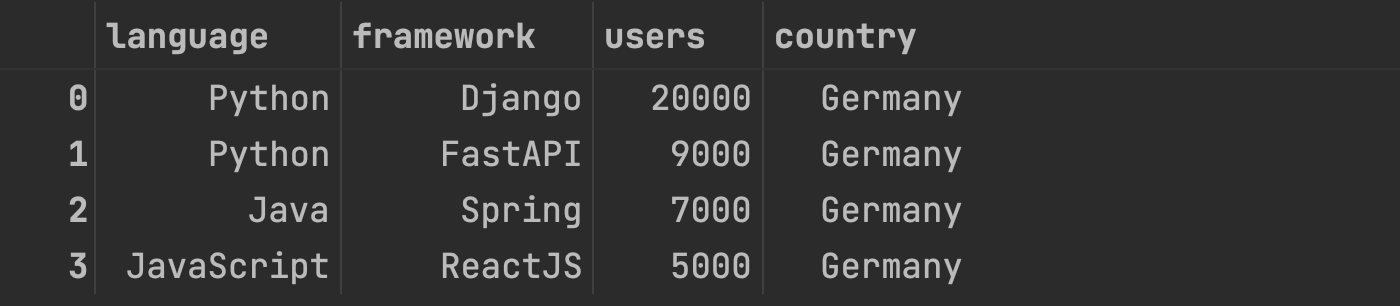
Add Column based on another Column
Next, we would like to add the column "user_percent" with the percentage of the existing column "users".
To do this, we use square brackets []
and the sum() method of Pandas:
df["users_percent"] = df["users"] / df["users"].sum()
df
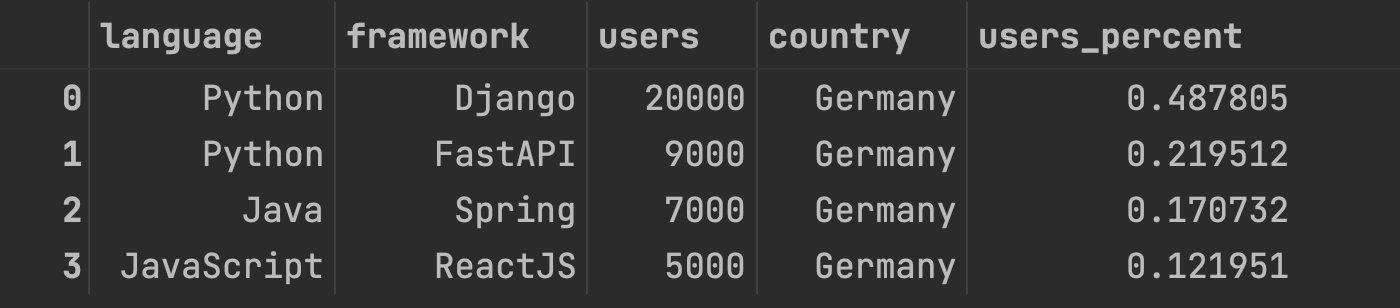
Add Column based on Condition
Next, we would like to add the column "type" with the values "Backend", "Frontend" and None depending on the values of column "language".
To do this, we use square brackets []
and the where() function of NumPy:
df["type"] = np.where(
df["language"].isin(["Python", "Java"]),
"Backend",
np.where(
df["language"] == "JavaScript",
"Frontend",
np.nan
)
)
df

Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to add columns to a Pandas DataFrame. We can simply use square brackets []
with the name of the new column. Try it yourself!