Introduction
In this tutorial, we want to show different ways how to create an array. In order to create an array, we use the functions array(), zeros(), arange() and linespace() of NumPy.
Import Libraries
First, we import the following python module:
import numpy as np
Array function
NumPy offers different possibilities to create an array. We can simply use the array() function to create a one-dimensional array from a list.
a = np.array([1,2,3,4,5])
print(a)
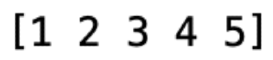
Zeros function
In order to create an array consisting of zeros, we can use the zeros() function. The first argument represents the shape of the array. We can also specify the data type of the values with the second argument dtype. In our example, we want to have integer values.
b = np.zeros(5, dtype=int)
print(b)
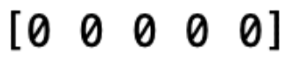
Ones function
In order to create a vector consisting of ones, we can use the ones() function. The first argument of the function represents the shape of the array. We can also specify the data type of the values with the second argument dtype. In our example, we want to have integer values.
c = np.ones(5, dtype=int)
print(c)
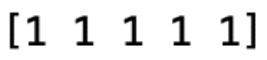
Arange function
In order to create an array consisting of numbers from a specified interval, we can use the arange() function. The first argument of the function is the start value of the interval. The end of the interval can be specified with the second argument (this value is not included). The third argument represents the step size of the interval [start, stop).
d = np.arange(1, 11, 2)
print(d)
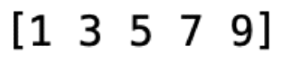
Linspace function
In order to create an array consisting of numbers from a specified interval, we can use the linspace() function. The first argument of the function is the start value of the interval. The end of the interval can be specified with the second argument (this value is included). The third argument num represents the number of samples of the interval [start, stop].
e = np.linspace(1, 2, num=5)
print(e)

Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen different ways how to create an array. We can simply use the functions array(), zeros(), arange() and linespace() of NumPy. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.