Introduction
In this tutorial, we want to create a Barplot. In order to do this, we use the barplot() function of Seaborn.
Import Libraries
First, we import the following python modules:
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
Load Data
We would like to use a seaborn sample dataset. Let's load the "tips" dataset into python.
tips_df = sns.load_dataset("tips")
tips_df.head()
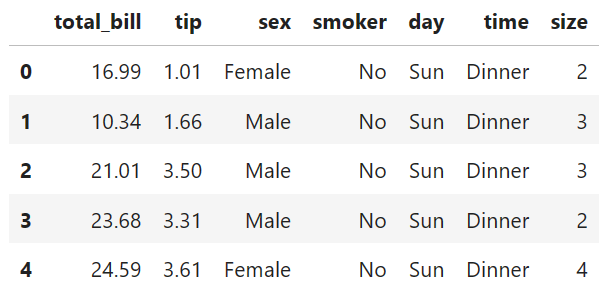
Barplot
In a Barplot, a categorical variable is used on one axis and a numerical variable on the other axis. The numerical values are aggregated for the specific categories. By default, the mean is taken as the aggregation function.
Example 1: Simple Barplot
We want to visualize the average tips per day with a Barplot. In order to do this, we set the x parameter to "day" and the y parameter to "tip". We use the color palette "crest".
sns.barplot(data=tips_df, x="day", y="tip",
palette="crest")
plt.show()
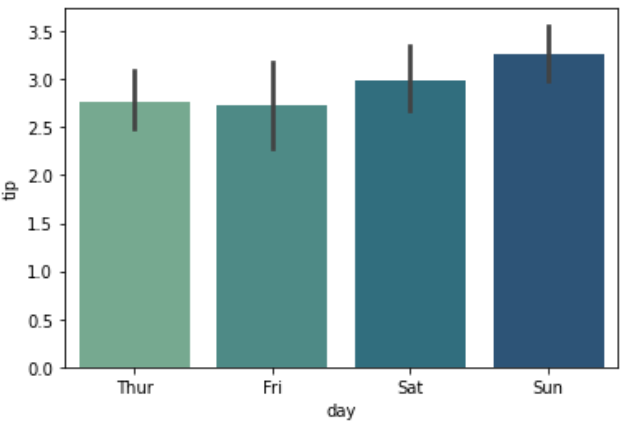
Example 2: Horizontal Barplot
Now, let's align the bars horiziontal instead of vertical. To do this, we swap the x and y axes.
sns.barplot(data=tips_df, x="tip", y="day",
palette="crest")
plt.show()
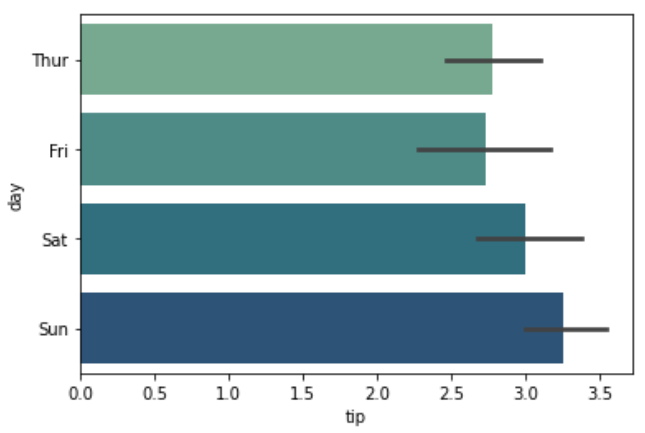
Example 3: Barplot with Grouping
Now, let's analyze the average tips per day for lunch and dinner. In order to do this, we use the hue parameter and set it to "time".
sns.barplot(data=tips_df, x="day", y="tip", hue="time",
palette="crest")
plt.show()
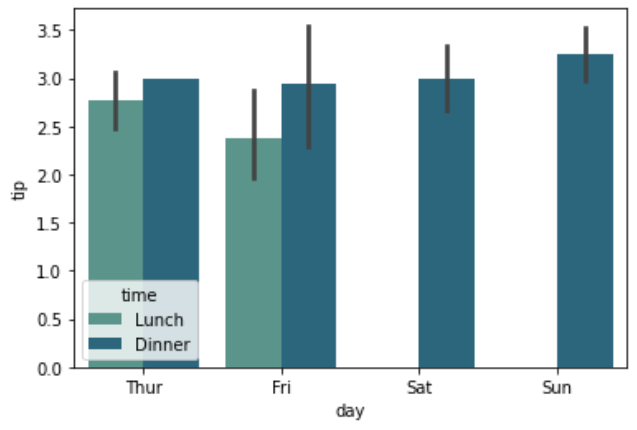
Example 4: Change Aggregation Function
Now, let's visualize the total tips per day. In order to do this, we use the estimator parameter and set it to np.sum. Here the sum() function from NumPy is used.
sns.barplot(data=tips_df, x="day", y="tip", estimator=np.sum,
palette="crest")
plt.show()
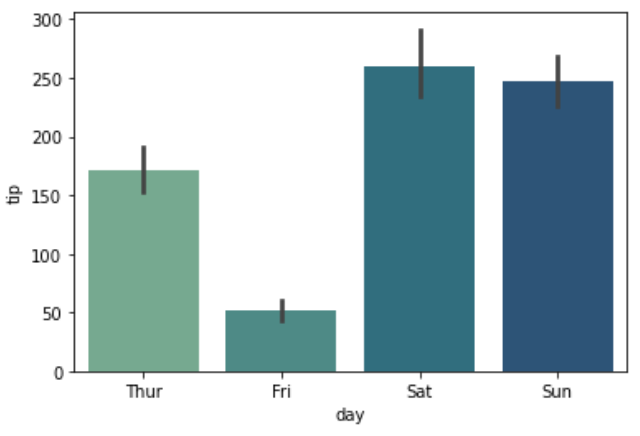
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to create a Barplot. We can simply use the barplot() function of Seaborn. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.