Introduction
In this tutorial, we want to write a Pandas DataFrame to an Excel file. In order to do this, we use the to_excel() method of Pandas.
Import Libraries
First, we import the following python modules:
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
data = {
"language": ["Python", "Python", "Java", "JavaScript"],
"framework": ["Django", "FastAPI", "Spring", "ReactJS"],
"users": [20000, 9000, 7000, 5000]
}
df = pd.DataFrame(data)
df
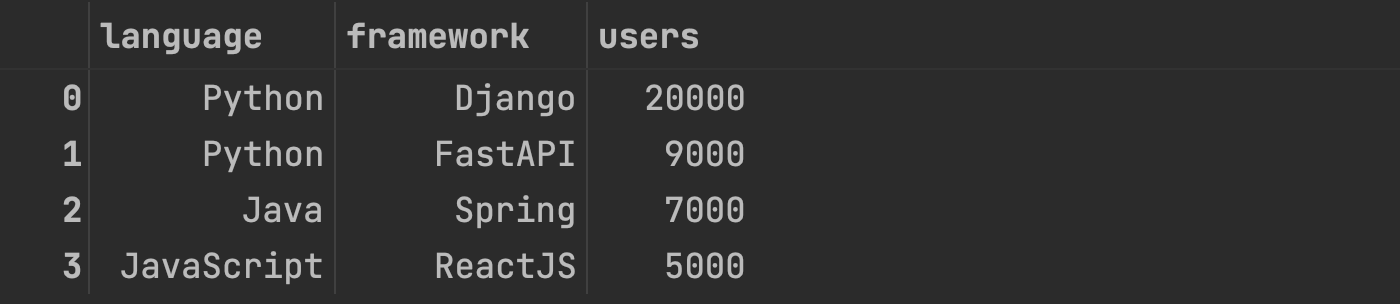
Write Pandas DataFrame to Excel File
Next, we would like to write the Pandas DataFrame to an Excel file. The file should have the following attributes:
- File should include a header with the column names.
- File should not include the index of the DataFrame.
- Sheet name should be Frameworks.
- File path should be "data/frameworks.xlsx".
To do this, we use the to_excel() method of Pandas:
df.to_excel("data/frameworks.xlsx", sheet_name="Frameworks", index=False)
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to write a Pandas DataFrame to an Excel file. We can simply use the to_excel() method of Pandas. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.