Introduction
In this tutorial, we want to sort a Pandas DataFrame by specific columns. In order to do this, we use the sort_values() method of Pandas.
Import Libraries
First, we import the following python modules:
import numpy as np
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
mydict = {
"column1": [3, 7, 7, 8, np.nan],
"column2": [11.3, 12.5, 19.2, 0.77, 9.4],
"column3": ["AI", "Python", "Python", np.nan, "AI"],
}
df = pd.DataFrame(mydict)
df
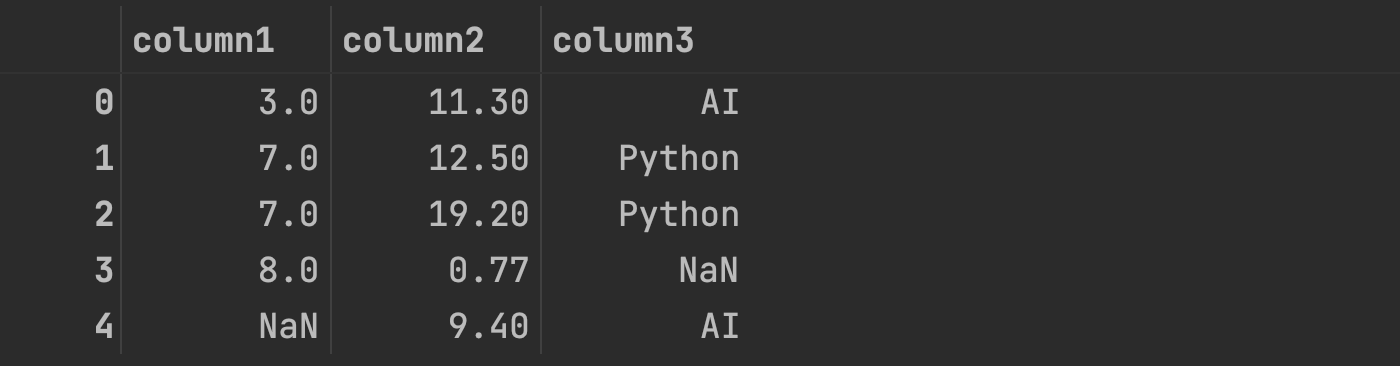
Sorting by a single Column
Ascending Order
We would like to sort the DataFrame by the column "column3" in ascending order.
To do this, we use the sort_values() method of Pandas. We pass the column name to sort by as argument and set the parameter "ascending" to True:
df_sorted = df.sort_values(
by="column3",
ascending=True
)
df_sorted
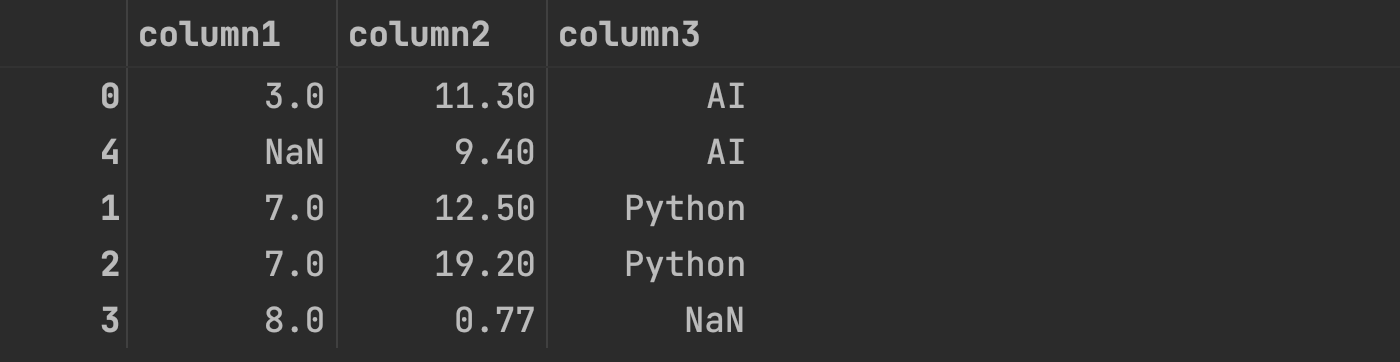
Descending Order
We would like to sort the DataFrame by the column "column3" in descending order.
To do this, we use the sort_values() method of Pandas. We pass the column name to sort by as argument and set the parameter "ascending" to False:
df_sorted = df.sort_values(
by="column3",
ascending=False
)
df_sorted
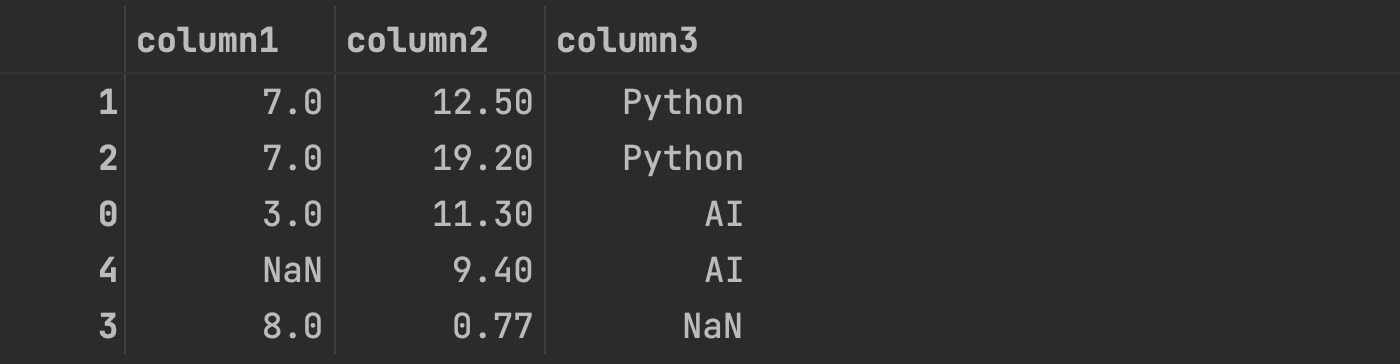
Sorting by multiple Columns
Now, we would like to sort the DataFrame by the column "column2" in ascending order and the column "column3" in descending order.
To do this, we use the sort_values() method of Pandas. We pass a list with the column names to sort by and a list with the boolean values identifing the orders as arguments:
df_sorted = df.sort_values(
by=["column2", "column3"],
ascending=[True, False]
)
df_sorted
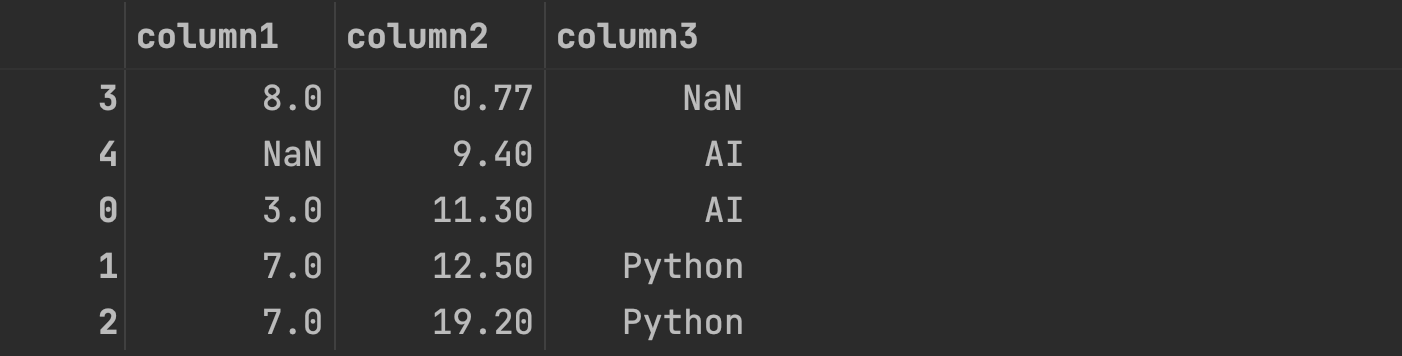
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to sort a Pandas DataFrame by specific columns. We can simply use the sort_values() method of Pandas. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.