Introduction
Data manipulation tasks often involve converting column data types to ensure consistency and accuracy in analysis. In this tutorial, we will show you how to change column types of a Pandas DataFrame. In order to do this, we will use the astype()
method, the map()
method and the to_datetime()
function of Pandas.
Import Libraries
First, we import the following python modules:
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
data = {
"language": ["Python", "Python", "Java", "JavaScript"],
"framework": ["Django", "FastAPI", "Spring", "ReactJS"],
"users": ["20000", "9000", "7000", "5000"],
"backend": ["true", "true", "true", "false"],
"date": ["2022-03-15", "2022-06-21", "2023-12-04", "2023-01-11"]
}
df = pd.DataFrame(data)
df
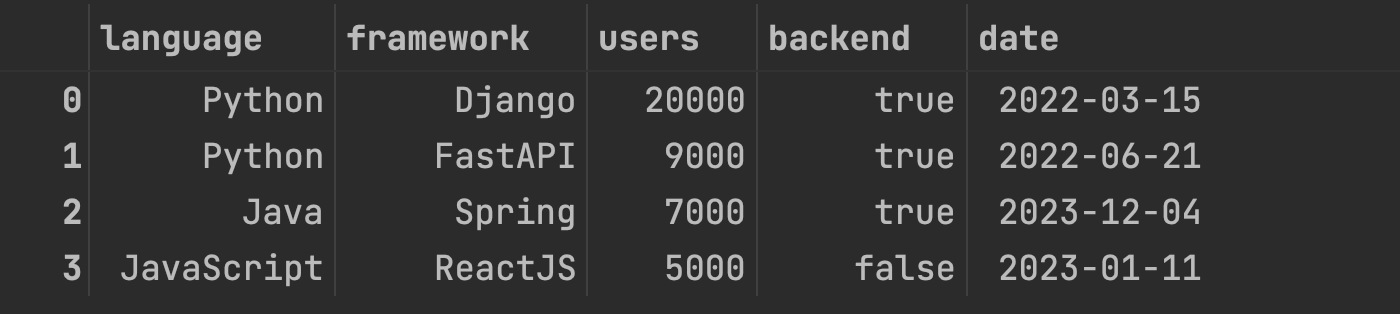
Let's print the schema of the DataFrame:
df.dtypes
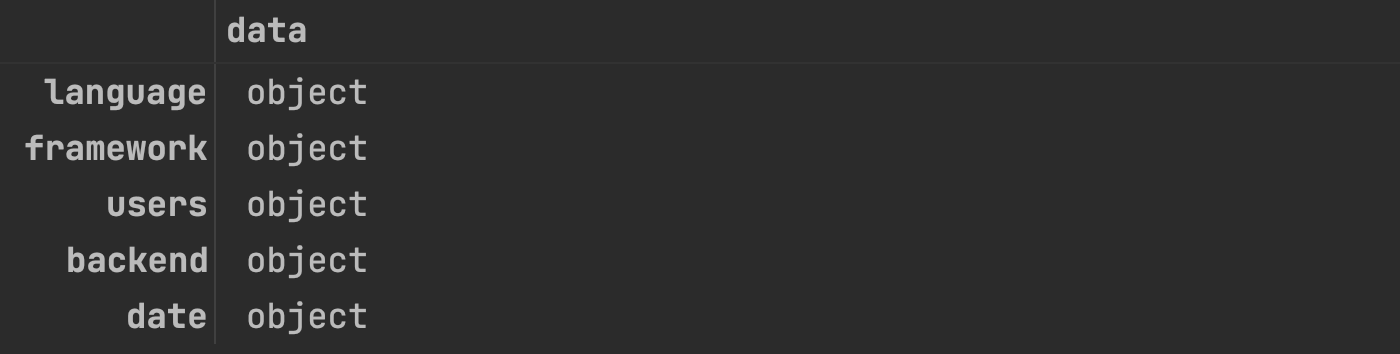
Change Data Type of a Single Column
Let's start with an example of converting the data type of a single column within a Pandas DataFrame.
We want to convert the data type of the column "users" from string
to integer
.
To do this, we use the astype()
method of Pandas:
# Convert string to integer
df["users"] = df["users"].astype(int)
# Print schema
df.dtypes
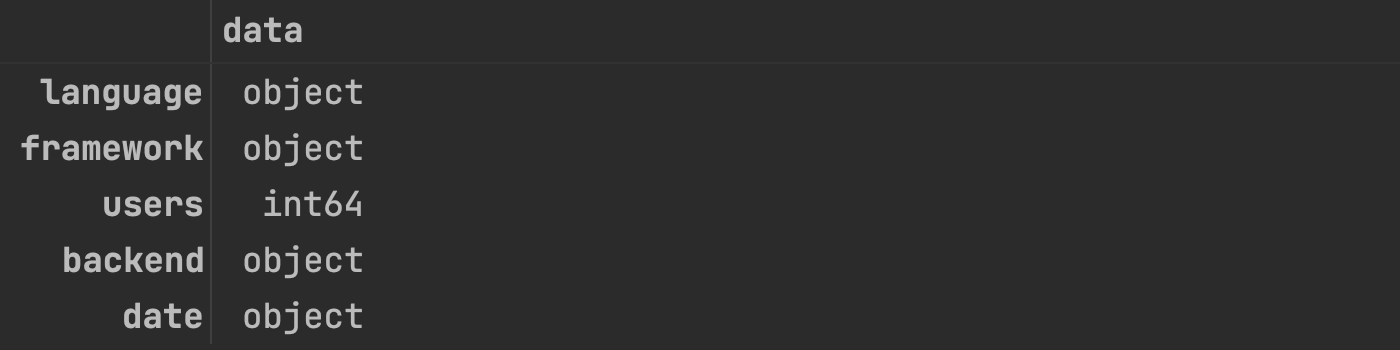
As you can see, the column "users" now has the desired data type.
Change Data Type of a Multiple Columns
Now, we want to change the data types of multiple DataFrame columns.
We want to do the following:
- Convert the data type of the column "users" from
string
tointeger
. - Convert the data type of the column "backend" from
string
toboolean
. - Convert the data type of the column "date" from
string
todatetime
.
To do this, we use the astype()
method, the map()
method and the to_datetime()
function of Pandas.
# Convert string to integer
df["users"] = df["users"].astype(int)
# Convert string to boolean
df["backend"] = df["backend"].map({"true": True, "false": False})
# Convert string to datetime
df["date"] = pd.to_datetime(df["date"])
# Print schema
df.dtypes
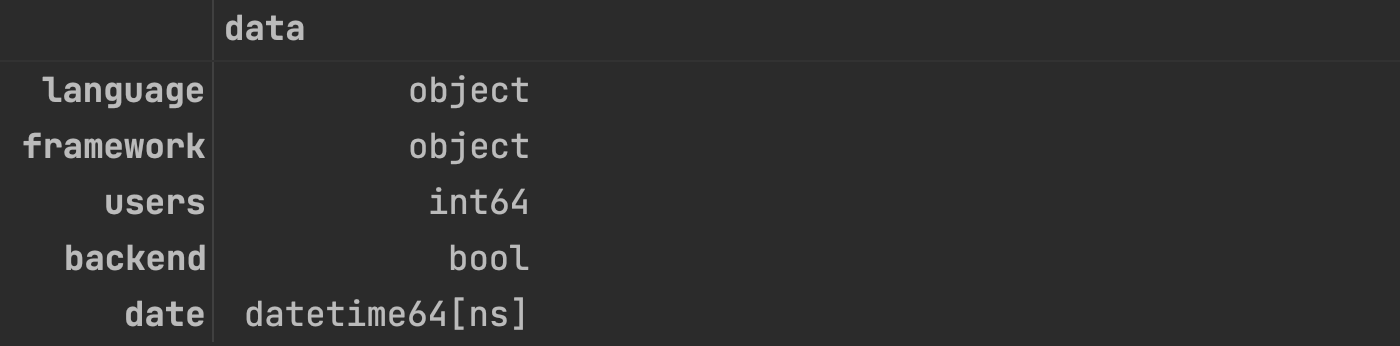
As you can see, the columns "users", "backend" and "date" now have the desired data types.
Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to change column types of a Pandas DataFrame. We can simply use the astype()
method, the map()
method and the to_datetime()
function of Pandas.. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.