Introduction
In this tutorial, we want to filter specific rows from a Pandas DataFrame based on specific conditions. In order to do this, we use DataFrame conditions with logical operators, the loc() method and the query() method of Pandas.
Import Libraries
First, we import the following python modules:
import pandas as pd
Create Pandas DataFrame
Next, we create a Pandas DataFrame with some example data from a dictionary:
data = {
"language": ["Python", "JavaScript", "Python", "Java"],
"framework": ["FastAPI", "ReactJS", "Django", "Spring"],
"users": [9000, 7000, 20000, 12000]
}
df = pd.DataFrame(data)
df
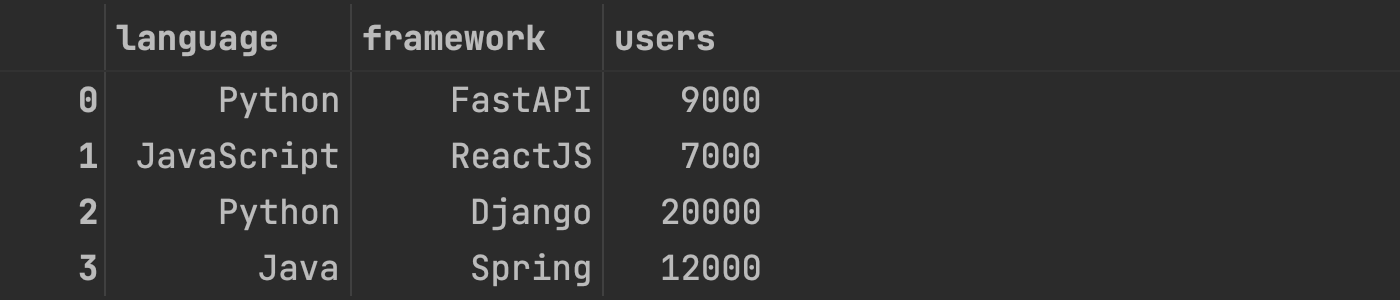
Filtering with Column Conditions
Now, we would like to filter the rows of the DataFrame based on multiple conditions.
To do this, we use logical operators on column values:
df_filtered = df[(df["language"] == "Python") & (df["users"] >= 10000)]
df_filtered

An alternative way is the following:
df_filtered = df[(df.language == "Python") & (df.users >= 10000)]
df_filtered

Besides, we get the same result by using the loc() method of Pandas:
df_filtered = df.loc[(df.language == "Python") & (df.users >= 10000)]
df_filtered

Filtering with Expression
Now, we would like to filter the rows of the DataFrame based on multiple conditions by using an expression.
To do this, we use the query() method of Pandas and pass the condition as a string:
df_filtered = df.query("language == 'Python' & users >= 10000")
df_filtered

Conclusion
Congratulations! Now you are one step closer to become an AI Expert. You have seen that it is very easy to filter specific rows from a Pandas DataFrame based on specific conditions. We can simply use DataFrame conditions with logical operators, the loc() method and the query() method of Pandas. Try it yourself!
Also check out our Instagram page. We appreciate your like or comment. Feel free to share this post with your friends.